Link web2 & web3 profiles in React, updated playground
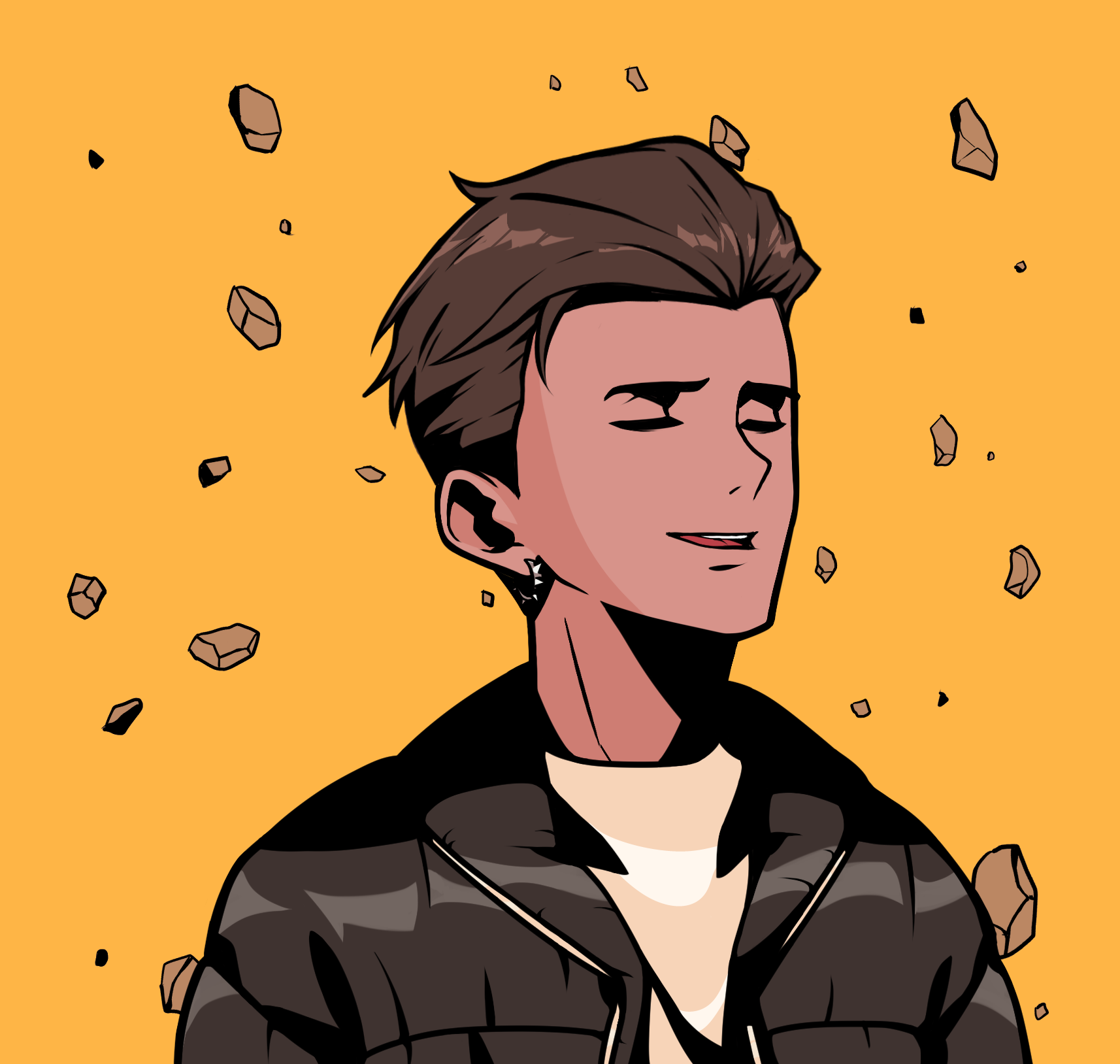
We shipped an update to make it easier to link multiple identities to the same in-app or ecosystem account on React web and native.
You can now use the useLinkProfiles()
to easily link a new identity to the connected account. A great use case for this is to onboard users with strategy: "guest"
to reduce friction, then when they've engaged with your app, prompt them to link an email or social account.
Once a user has linked an identity, they can use that identity to login back to the same account later. So in the example above, the user can now login with google next time they need to login to your app.
Another great feature is linking web3 wallets to the account:
This will require a signature, ensuring the user owns that wallet. A great use case is doing read only operations on the linked wallets, like checking social profiles, ownership of an NFT or onchain history.
You can fetch all the linked profiles using the useProfiles()
hook.
We also update the playground site, see it in action here: https://playground.thirdweb.com/connect/in-app-wallet
You can also view more detailed documentation on our developer portal.
Happy building! 🛠️